I have been experimenting with vertexes, and have come to simulate 3rd, 2nd, and 1st person view. I'm having trouble with a piece of the algorithm though.
I need to clip the image from view if it is NOT in front of the player IF the view is 1st person (line12)
Clipping the image out of view under this circumstance isn't too hard, though it includes the Cross Vector Product algorithm(line181), and I don't fully understand its black magic, yet.
The issue is the 2 of the arguments I need to pass are -0.0001, and 0.0001(line109-113), but I've become unsuccessful in trying to work around this. Perhaps I'm just tired.
YES ITS COMMENTED
Code: Select all
@echo off & setlocal enableDelayedExpansion
if "%~1" neq "" goto :%~1
REM INITIALIZE -----------------------------------------------------------------------------
call :macros
title Vertex rotation test
mode 100,100
REM CHANGE ME TO VALUE ( 1 - 1st Person; 2 - 2nd Person; 3 - 3rd Person )
set /a "view=1"
REM -----------------------------------------------------
rem The coordinates of the player
set /a "px=45", "py=45", "P_ANGLE=0"
rem The end coordinates for the line segment representing a "wall"
set /a "vx1=70", "vy1=20"
set /a "vx2=70", "vy2=70"
REM ----------------------------------------------------------------------------------------
if exist "%temp%\%~n0_signal.txt" del "%temp%\%~n0_signal.txt"
"%~F0" Controller WASDKL >"%temp%\%~n0_signal.txt" | "%~F0" ENGINE <"%temp%\%~n0_signal.txt"
:ENGINE
for /l %%# in () do ( set /a "frame+=1"
rem User Controller
set "com=" & set /p "com="
%= Calculate movement =%
if /i "!com!" equ "s" ( set /a "px-=3 * !cos(x):x=P_ANGLE!", "py-=3 * !sin(x):x=P_ANGLE!"
) else if /i "!com!" equ "w" ( set /a "px+=3 * !cos(x):x=P_ANGLE!", "py+=3 * !sin(x):x=P_ANGLE!"
) else if /i "!com!" equ "a" ( set /a "px-=3 * !sin(x):x=P_ANGLE!", "py-=3 * !cos(x):x=P_ANGLE!"
) else if /i "!com!" equ "d" ( set /a "px+=3 * !sin(x):x=P_ANGLE!", "py+=3 * !cos(x):x=P_ANGLE!"
) else if /i "!com!" equ "k" ( set /a "P_ANGLE-=10"
) else if /i "!com!" equ "l" ( set /a "P_ANGLE+=10"
)
REM BUILD VIEW ----------------------------------------------------------------------------
%= lx ly are the end of the player pointer =%
set /a "lx=7 * !cos(x):x=P_ANGLE! + px", "ly=7 * !sin(x):x=P_ANGLE! + py"
%= Transform the vertexes relative to the player =%
set /a "tx1=vx1 - px", "ty1=vy1 - py"
set /a "tx2=vx2 - px", "ty2=vy2 - py"
%= Rotate them around the players view =%
set /a "tz1=tx1 * !cos(x):x=P_ANGLE! + ty1 * !sin(x):x=P_ANGLE!"
set /a "tz2=tx2 * !cos(x):x=P_ANGLE! + ty2 * !sin(x):x=P_ANGLE!"
set /a "tx1=tx1 * !sin(x):x=P_ANGLE! - ty1 * !cos(x):x=P_ANGLE!"
set /a "tx2=tx2 * !sin(x):x=P_ANGLE! - ty2 * !cos(x):x=P_ANGLE!"
%= Translate into view =%
set /a "sz1=50 - tz1", "sz2=50 - tz2", "sx1=50 - tx1", "sx2=50 - tx2"
REM BUILD VIEW ----------------------------------------------------------------------------
if !view! equ 3 (
%= Draw the Absolute Map =%
%= 3rd Person View =%
%line% %vx1% %vy1% %vx2% %vy2% Û 11
%line% !px! !py! !lx! !ly! Û 255
%fastCircle% !px! !py! 2 2 330
%= ----------------------------------------------------------------------------------- =%
) else if !view! equ 2 (
%= Draw the Transformed Map =%
%= 2nd Person View =%
%line% !sx1! !sz1! !sx2! !sz2! Û 11
%line% 50 50 50 43 Û 255
%fastCircle% 50 50 2 2 330
%= ----------------------------------------------------------------------------------- =%
) else if !view! equ 1 (
if !tz1! gtr 0 ( set "b1=0" ) else ( set "b1=1" )
if !tz2! gtr 0 ( set "b2=0" ) else ( set "b2=1" )
set /a "bool=(b1|b2)"
REM IMPORTANT!!!
rem All commented code here is the Cross Vector Product Algorithm in the works.
rem including INTERSECT macro
REM if !bool! equ 1 (
rem v-------------------{ These values SHOULD be (according to the algorithm)
rem v-----------------{ -0.0001 0.0001
%intersect% !tx1! !tz1! !tx2! !tz2! -1 1 -20 5 ix1 iz1
rem v-------------------{ These values SHOULD be (according to the algorithm)
rem v-----------------{ 0.0001 0.0001
%intersect% !tx1! !tz1! !tx2! !tz2! 1 1 20 5 ix2 iz2
REM if !tz1! leq 0 if !iz1! gtr 0 ( set /a "tx1=ix1", "tz1=iz1" ) else ( set /a "tx1=ix2", "tz1=iz2")
REM if !tz2! leq 0 if !iz1! gtr 0 ( set /a "tx2=ix1", "tz2=iz1" ) else ( set /a "tx2=ix2", "tz2=iz2")
%= Draw the Perspective-Transformed Map =%
set /a "x1=-tx1 * 16 / tz1", "y1a=-50 / tz1", "y1b=50 / tz1"
set /a "x2=-tx2 * 16 / tz2", "y2a=-50 / tz2", "y2b=50 / tz2"
%= Translate into view =%
for %%a in (x1 x2 y1a y1b y2a y2b) do set /a "%%a=50 + %%a"
%= 1st Person View =%
%= top (1-2 b) =%
%line% !x1! !y1a! !x2! !y2a! Û 11
%= bottom (1-2 b) =%
%line% !x1! !y1b! !x2! !y2b! Û 11
%= left (1) =%
%line% !x1! !y1a! !x1! !y1b! Û 9
%= right (2) =%
%line% !x2! !y2a! !x2! !y2b! Û 9
REM )
%= ----------------------------------------------------------------------------------- =%
)
rem display everything using VT100 2J sequence to clear the screen first.
<nul set /p "=%ESC%[2J!screen!" & set "screen="
)
exit
:Controller
( for /l %%# in () do ( for /f "tokens=*" %%a in ('choice /c:%~2 /n') do ( <nul set /p ".=%%a" ) ) )
:macros
set ^"LF=^
^" Above empty line is required - do not remove
set ^"\n=^^^%LF%%LF%^%LF%%LF%^^"
for /F %%a in ('echo prompt $E^| cmd') do set "ESC=%%a"
<nul set /p "=!esc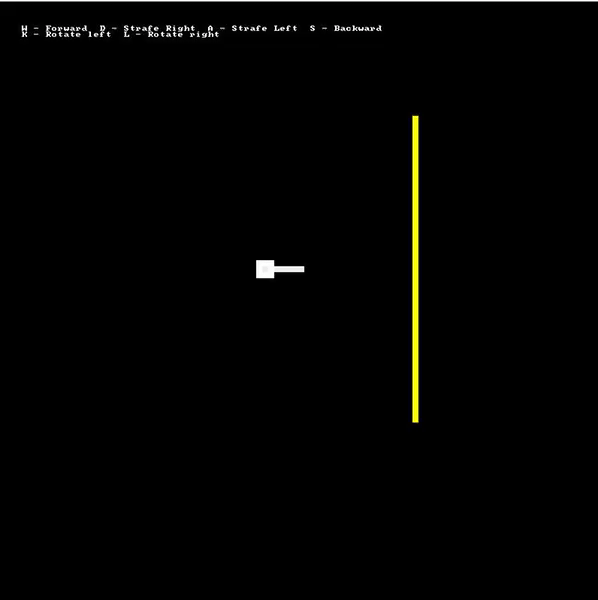
2nd person
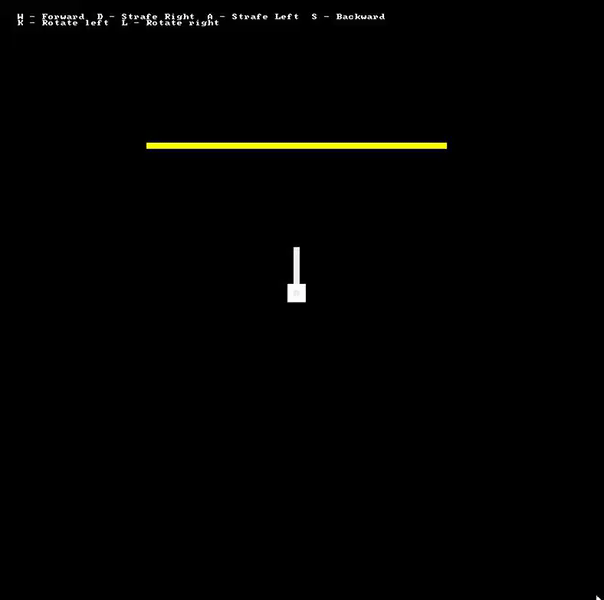
1st person
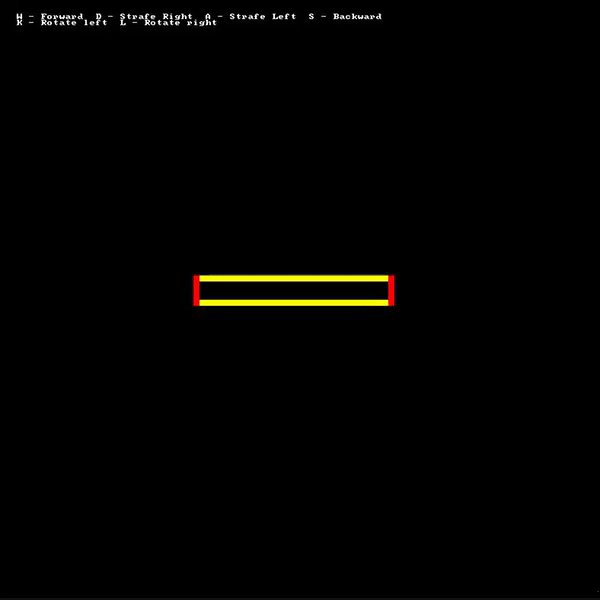